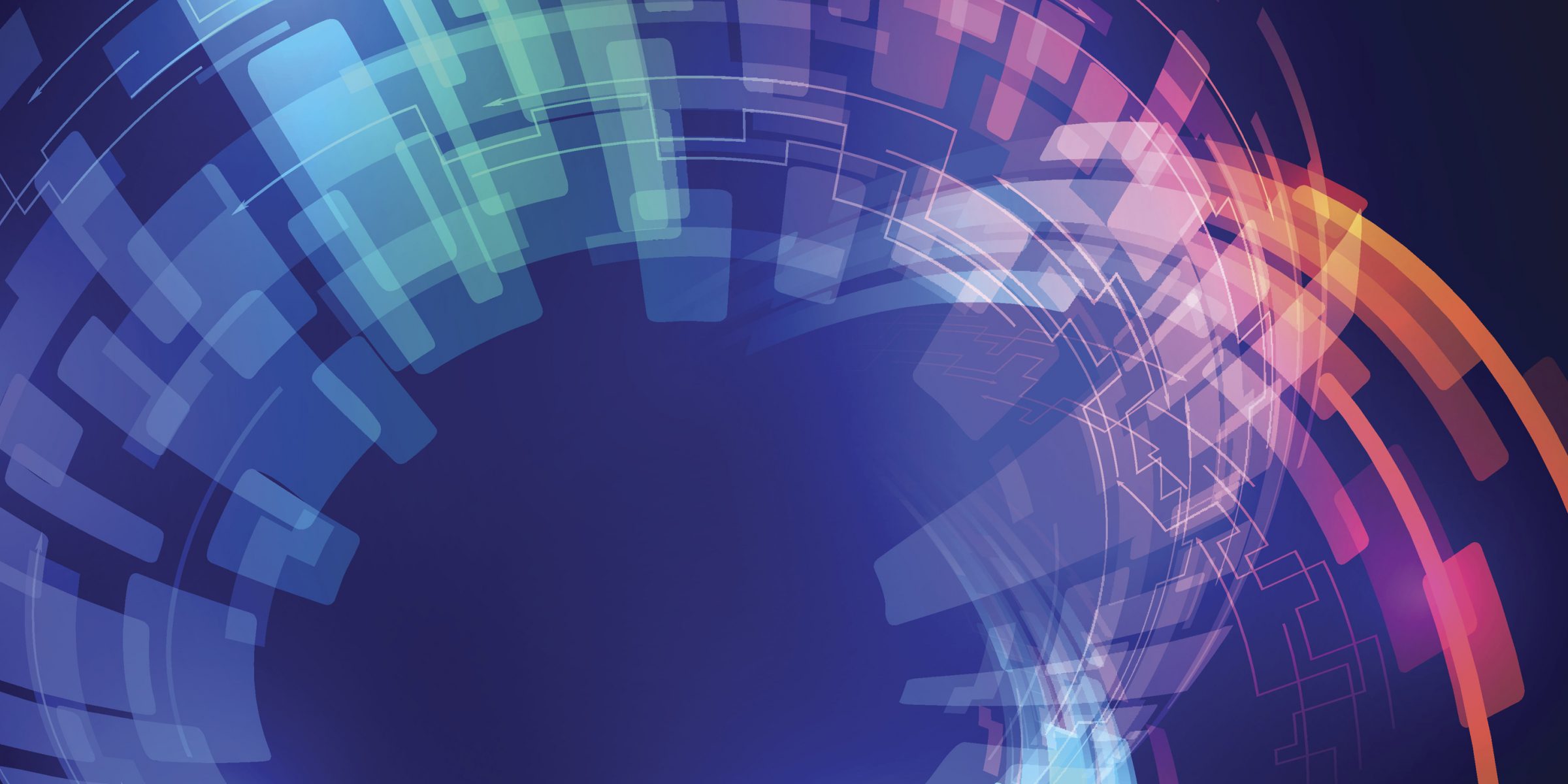
Ada for micro:bit Part 5: Analog Output
by Fabien Chouteau –
Welcome to the Ada for micro:bit series where we look at simple examples to learn how to program the BBC micro:bit with Ada.
If you haven't already, please follow the instructions in Part 1 to setup your development environment.
In this fifth part we will see how to write an analog value to a pin. The micro:bit doesn't have a real digital to analog converter, so the analog signal is actually a Pulse Width Modulation (PWM). This is good enough to control the speed of a motor or the brightness of an LED.
There is a limit of three analog (PWM) signals on the micro:bit, if you try to write an analog value to more than three pins an exception will be raised.
Wiring Diagram
For this example we use the same circuit as the pin output example.
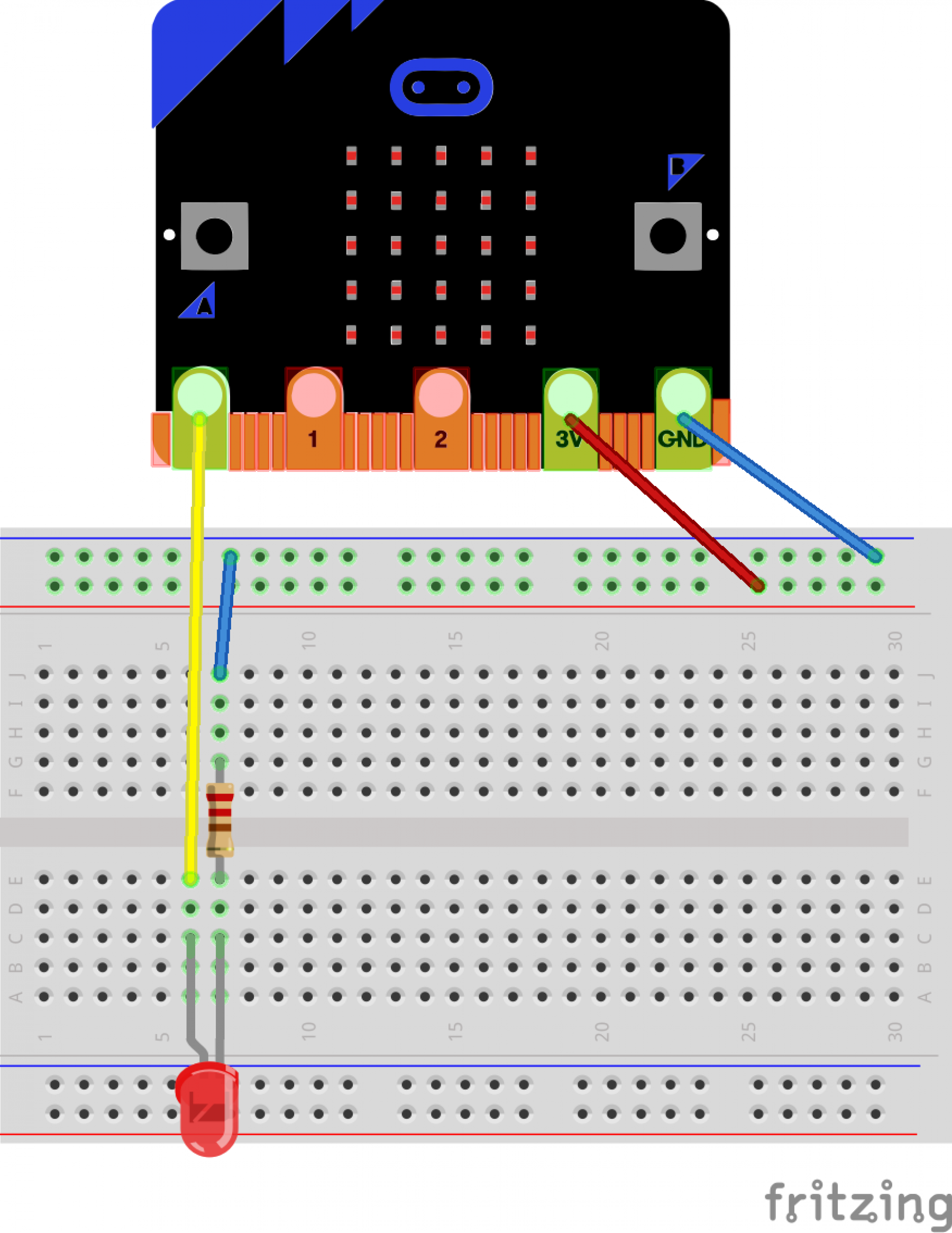
Interface
To write an analog value to the IO pin we are going to use the procedure Write
of the package MicroBit.IOs
.
procedure Write (Pin : Pin_Id; Value : Analog_Value)
with Pre => Supports (Pin, Analog);
Arguments:
- Pin : The id of the pin that we want to read as digital input
- Value : The analog value for the pin, between 0 and 1023
Precondition:
- The procedure
Write
has a precondition that the pin must support analog IO.
In the code, we are going to write an loop with a value that goes from 0 to 128 and set write this value to pin 0. We could go from 0 to 1023 but since the LED doesn't get brighter after 128, there is no need to go beyond that value.
We also use the procedure Delay_Ms
of the package MicroBit.Time
to stop the
program for a short amount of time.
Here is the full code of the example:
with MicroBit.IOs;
with MicroBit.Time;
procedure Main is
begin
-- Loop forever
loop
-- Loop for value between 0 and 128
for Value in MicroBit.IOs.Analog_Value range 0 .. 128 loop
-- Write the analog value of pin 0
MicroBit.IOs.Write (0, Value);
-- Wait 20 milliseconds
MicroBit.Time.Delay_Ms (20);
end loop;
end loop;
end Main;
Following the instructions of Part 1 you can open this example (Ada_Drivers_Library-master\examples\MicroBit\analog_out\analog_out.gpr), compile and program it on your micro:bit.
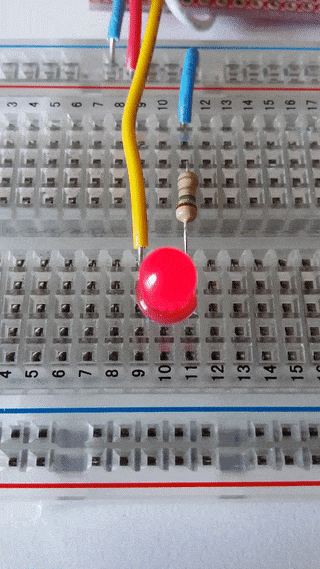
See you next week for another Ada project on the micro:bit.
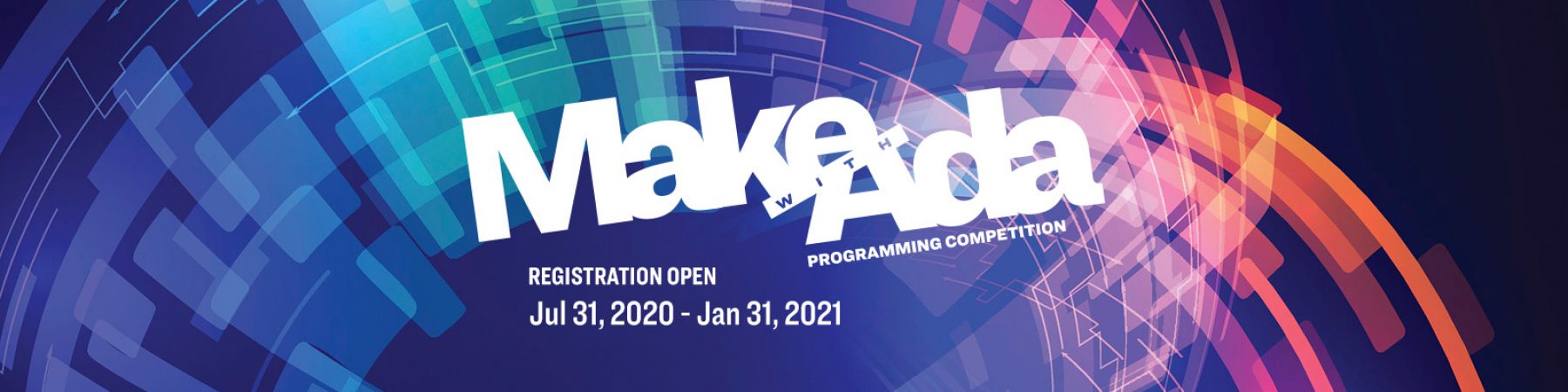
Don't miss out on the opportunity to use Ada in action by taking part in the fifth annual Make with Ada competition! We're calling on developers across the globe to build cool embedded applications using the Ada and SPARK programming languages and are offering over $9,000 in total prizes. Find out more and register today!