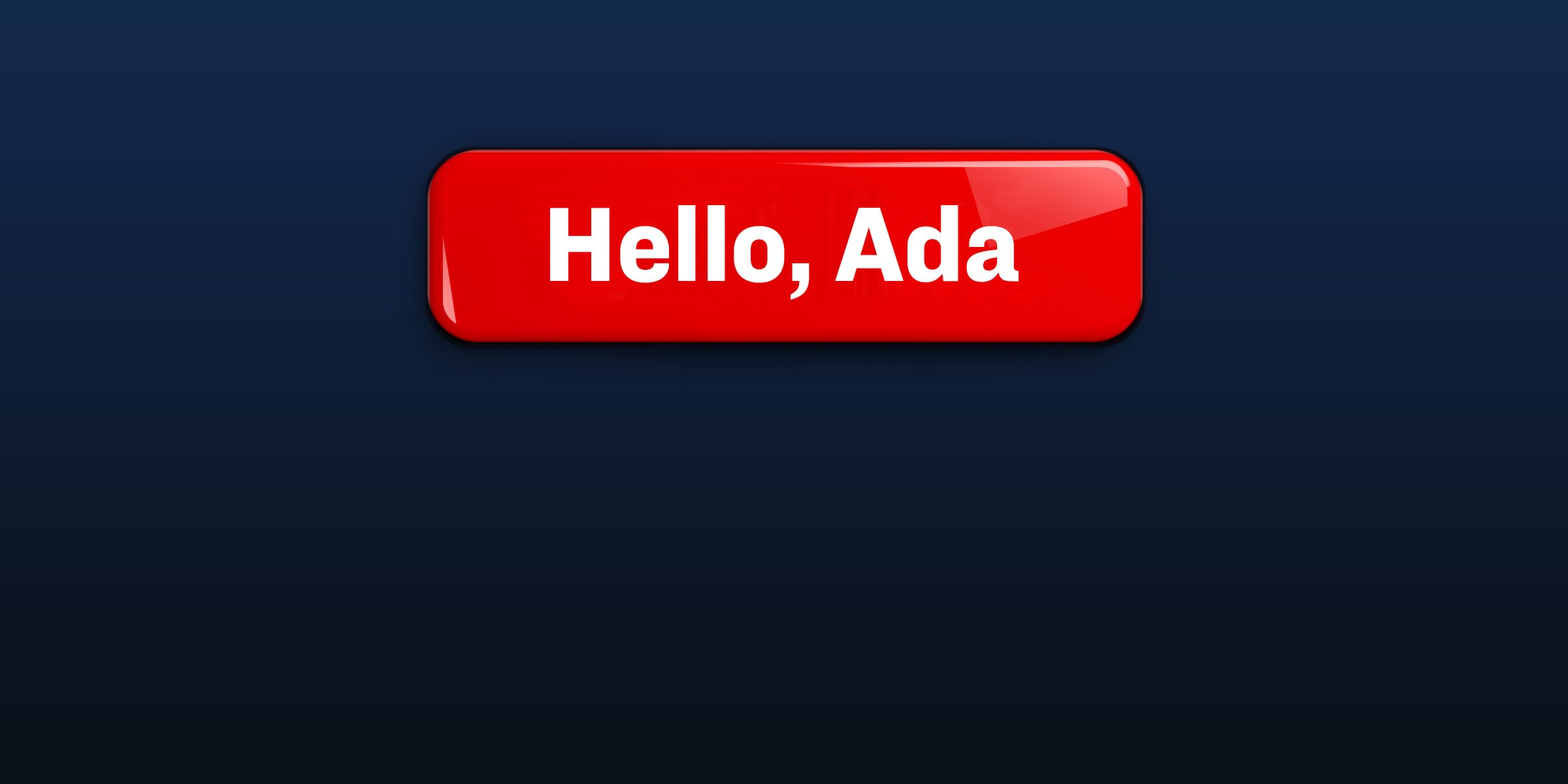
Using GNAT-LLVM to target Ada to WebAssembly
by Vadim Godunko –
The GNAT-LLVM project provides an opportunity to port Ada to new platforms, one of which is WebAssembly. We conducted an experiment to evaluate the porting of Ada and the development of bindings to use Web API provided by the browser directly from Ada applications.
Subtotals
As a result of the experiment, the standard language library and runtime library were partially ported. Together with a binding for the Web API, this allowed us to write a simple example showing the possibility of using Ada for developing applications compiled into WebAssembly and executed inside the browser. At the same time, there are some limitations both of WebAssembly and of the current GNAT-LLVM implementation:
- the inability to use tasks and protected types
- support for exceptions limited to local propagation and the last chance handler
- the inability to use nested subprograms
Example
Here is small example of an Ada program that shows/hides the text when pressing the button by manipulating attributes of document nodes.
with Web.DOM.Event_Listeners;
with Web.DOM.Events;
with Web.HTML.Buttons;
with Web.HTML.Elements;
with Web.Strings;
with Web.Window;
package body Demo is
function "+" (Item : Wide_Wide_String) return Web.Strings.Web_String
renames Web.Strings.To_Web_String;
type Listener is
limited new Web.DOM.Event_Listeners.Event_Listener with null record;
overriding procedure Handle_Event
(Self : in out Listener;
Event : in out Web.DOM.Events.Event'Class);
L : aliased Listener;
------------------
-- Handle_Event --
------------------
overriding procedure Handle_Event
(Self : in out Listener;
Event : in out Web.DOM.Events.Event'Class)
is
X : Web.HTML.Elements.HTML_Element
:= Web.Window.Document.Get_Element_By_Id (+"toggle_label");
begin
X.Set_Hidden (not X.Get_Hidden);
end Handle_Event;
---------------------
-- Initialize_Demo --
---------------------
procedure Initialize_Demo is
B : Web.HTML.Buttons.HTML_Button
:= Web.Window.Document.Get_Element_By_Id
(+"toggle_button").As_HTML_Button;
begin
B.Add_Event_Listener (+"click", L'Access);
B.Set_Disabled (False);
end Initialize_Demo;
begin
Initialize_Demo;
end Demo;
As you can see, it uses elaboration, tagged and interface types, and callbacks.
Live demo
Hello, World!
Setup & Build
To compile the examples you need to setup GNAT-LLVM & GNAT WASM RTL following instructions in README.md file.
To compile specific example use gprbuild to build application and open index.html in the browser to run it.
Next steps
The source code is published in a repository on GitHub and we invite everyone to participate in the project.